In the world of modern cloud computing and infrastructure management, the need for automation, scalability, and consistency has never been greater. Infrastructure as Code (IaC) tools, such as Terraform, have emerged as the solution to these challenges. This article provides a comprehensive introduction to Terraform, its key concepts, and how it revolutionizes the way we manage infrastructure.
What is Terraform?
Terraform is an open-source Infrastructure as Code (IaC) tool created by HashiCorp. It allows you to define and provision infrastructure resources using a declarative configuration language. With Terraform, you describe the desired state of your infrastructure in code, and Terraform takes care of creating, updating, and managing the resources necessary to achieve that state.
Why use Terraform?
- Automation
- Terraform automates the provisioning and management of infrastructure, reducing manual tasks and human errors.
- Scalability
- As your infrastructure needs grow, Terraform can scale with you, ensuring consistent provisioning across environments.
- Consistency
- Terraform ensures that infrastructure remains consistent across development, testing, and production environments.
- Collaboration
- Teams can collaborate on infrastructure changes using version control systems like Git, tracking changes and enabling code reviews.
Key features in Terraform
Declarative Configurations
Terraform uses a declarative configuration language, HashiCorp Configuration Language (HCL), to define infrastructure. Instead of specifying the step-by-step process of provisioning resources, you declare the desired outcome, and Terraform handles the details.
Resource and Provider Model
Resources represent infrastructure components (e.g., virtual machines, networks, databases), and providers are responsible for managing those resources within a specific cloud or service (e.g., AWS, Azure, Docker).
State Management
Terraform maintains a state file that records the current state of your infrastructure. This state allows Terraform to identify what changes need to be made when you update your configuration.
Getting Stated with Terraform
Installation
To get started with Terraform, you need to download and install it on your system. Terraform provides binaries for various platforms, making it accessible on most operating systems. You can download Terraform form official website.
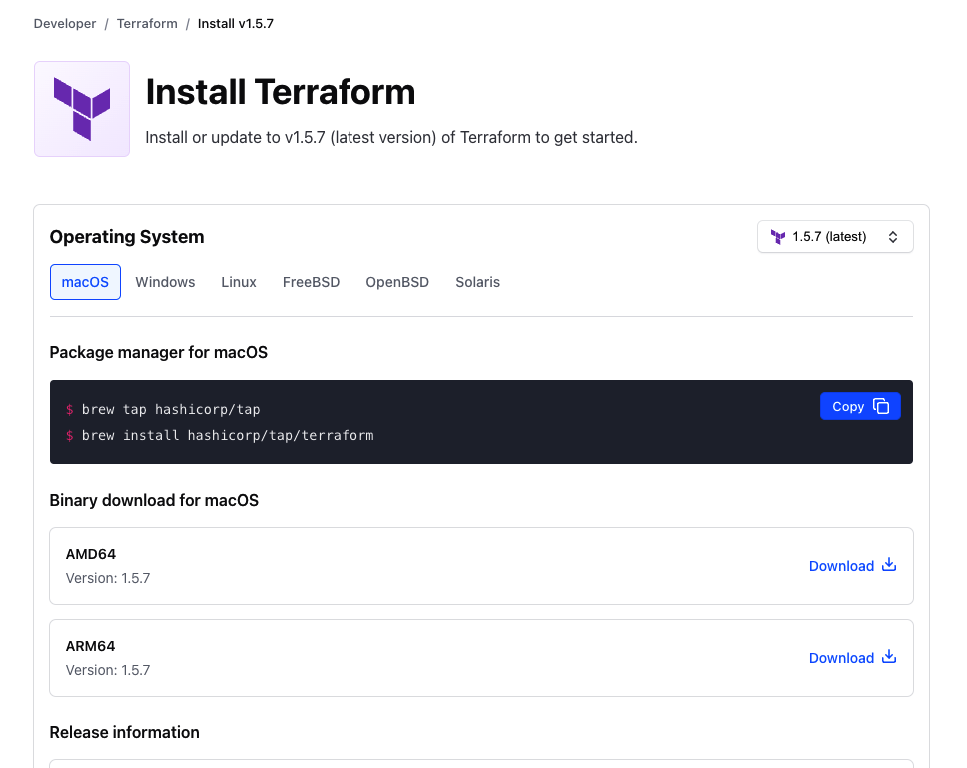
Writing your first configuration
Terraform configurations are written in HCL. You define resources, providers, and configurations in .tf
files. A simple configuration might define an AWS EC2 instance, specifying the instance type, AMI, and other parameters.
# Define the AWS provider and region
provider "aws" {
region = "us-east-1" # Change this to your desired AWS region
}
# Define an AWS EC2 instance
resource "aws_instance" "example" {
ami = "ami-0c55b159cbfafe1f0" # Replace with your desired AMI ID
instance_type = "t2.micro" # Set the instance type (e.g., t2.micro)
}
# Output the public IP address of the created instance
output "public_ip" {
value = aws_instance.example.public_ip
}
Initializing and apply configurations
After writing your configuration, you run terraform init
to initialize your working directory and download necessary providers. Then, use terraform apply
to create or update infrastructure based on your configuration.
Terraform Language and Syntax
- Variables and Expressions
- Terraform supports variables and expressions, allowing you to parameterize your configurations. This enables reusability and dynamic configuration.
- Modules
- Modules in Terraform allow you to organize and reuse configurations. They encapsulate resources and configurations, making it easier to manage complex infrastructure.
- Functions
- Terraform includes a set of built-in functions for manipulating and transforming data within your configurations. Functions enhance configuration flexibility.
Working with Terraform
- Managing Resources
- Terraform provides commands like
terraform plan
andterraform apply
to manage resources. Theplan
command shows you what changes Terraform will make before applying them.
- Terraform provides commands like
- State and State Files
- The Terraform state file is critical for tracking your infrastructure. You can store the state locally or remotely, depending on your requirements.
- Terraform commands
- Terraform offers a wide range of commands, including
validate
,destroy
, andimport
, to manage your infrastructure.
- Terraform offers a wide range of commands, including
Terraform in Real-World scenarios
- Infrastructure provisioning
- Terraform is used extensively for provisioning cloud resources, such as virtual machines, storage, and networks.
- Multi Environment management
- Terraform can manage infrastructure across different environments, including development, testing, staging, and production.
- Collaboration and Version control
- Teams collaborate on Terraform configurations using version control systems like Git, ensuring changes are tracked, reviewed, and audited.
Best Practices in Terraform
- Organizing Terraform code
- Best practices include structuring your Terraform code into meaningful directories, using naming conventions, and separating configurations by environment.
- Managing secrets
- Securely manage secrets and sensitive data using Terraform variables, environment variables, or external secrets management solutions.
- Testing infrastructure as code
- Implement testing practices to ensure that your Terraform configurations are valid, maintainable, and reliable.
Security and Compliance
- Security considerations
- Terraform users should follow security best practices, such as role-based access control (RBAC), to protect infrastructure configurations.
- Compliance as code
- Terraform can help enforce compliance policies by codifying security and compliance requirements.
Scaling
Large scale deployments
Managing large-scale deployments with Terraform involves careful planning and optimization.
- Modularization
- Break down your Terraform code into smaller, reusable modules. This simplifies management and encourages code reuse.
- Remote state storage
- Use remote state storage solutions, such as Amazon S3 or HashiCorp Consul, to centralize your state files. This allows for collaboration and locking when multiple team members work on the same configuration.
- Parallel execution
- Terraform can apply changes in parallel using the
-parallelism
flag, allowing you to speed up the provisioning of resources.
- Terraform can apply changes in parallel using the
Remote state storage
As mentioned earlier, using remote state storage is crucial for large-scale deployments. It centralizes your state files, making them accessible to your entire team. Terraform offers various backends for remote state storage, including Amazon S3, Google Cloud Storage, and HashiCorp Consul. Configuring remote state involves updating your backend configuration in your Terraform code. Here’s an example of configuring remote state storage with Amazon S3.
terraform {
backend "s3" {
bucket = "my-terraform-state-bucket"
key = "path/to/my/terraform.tfstate"
region = "us-east-1"
encrypt = true
dynamodb_table = "my-lock-table"
}
}
Terraform cloud and Enterprise
For organizations with more extensive infrastructure requirements, HashiCorp offers Terraform Cloud and Terraform Enterprise. These solutions provide collaboration features, policy as code, and advanced governance capabilities. They also support integration with version control systems and offer secure and scalable environments for Terraform workflows.
Challenges and Limitations
State Locking
Terraform uses state files to track the current state of your infrastructure. When multiple team members or automation processes modify the same state file simultaneously, it can lead to conflicts and inconsistencies. To address this, Terraform provides state locking mechanisms, such as DynamoDB locks for Amazon S3 backend. Properly configuring state locking is essential for avoiding data corruption and conflicts in a team environment.
Handling sensitive data
Managing sensitive data, such as passwords, API keys, or certificates, in Terraform can be challenging. It’s crucial to avoid hardcoding sensitive information in your configuration files. Instead, consider using external secret management tools like HashiCorp Vault or AWS Secrets Manager. These tools allow you to securely manage and inject sensitive data into your infrastructure.
Immutable Infrastructure
Terraform is designed for managing infrastructure as code and follows the immutable infrastructure paradigm. This means that when you want to make changes to your infrastructure, Terraform doesn’t modify the existing resources; it creates new ones and destroys the old ones. While this approach has many benefits, it can also lead to challenges when dealing with stateful resources or complex migration scenarios.
Terraform is a powerful Infrastructure as Code tool that simplifies infrastructure provisioning and management. By following best practices, embracing advanced topics like large-scale deployments and remote state storage, and addressing challenges and limitations, you can effectively leverage Terraform to automate and scale your infrastructure.
Thanks for sharing Gayan !