ChatGPT, developed by OpenAI, is a state-of-the-art language model that can generate human-like text responses. It’s a powerful tool that can be used to build chatbots, virtual assistants, and even simulate conversations with AI. In this article, I will walk you through creating a simple “Hello World” chatbot using ChatGPT and Python.
Prerequisites
Before you dive into building ChatGPT powered application, you need to have following prerequisites.
- Python: Make sure you have Python 3.x installed on your machine.
- IDE or Text Editor: You will require an IDE or Text Editor to edit source files.
STEP 1: Obtain OpenAI API key
Open a web browser and Navigate into https://platform.openai.com. Then you will required to sign-in or sign-up. Once you landed on the Open AI platform playground navigate into account then API Keys section.
Once you navigate into API Keys section you can generate new key using Create new secret key button.
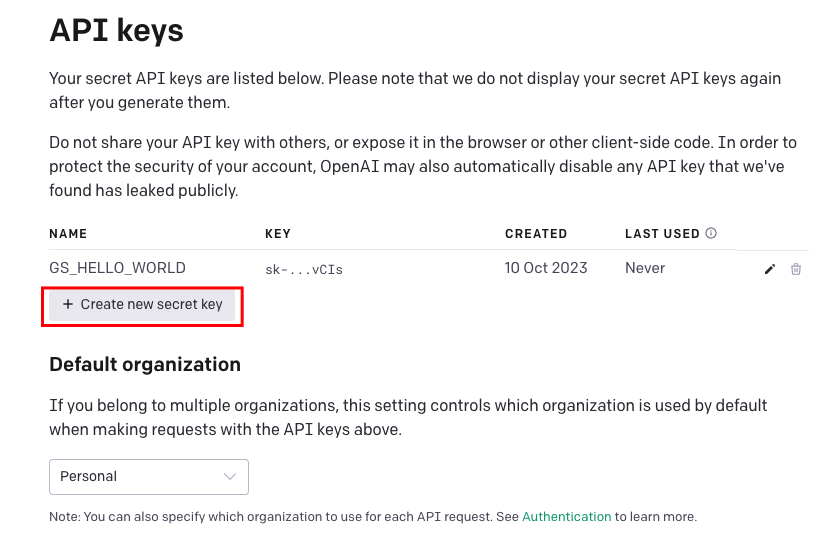
Copy the generated secret key and use it in the following code that we going to implement.
STEP 2: Setting up Python Environment
Navigate to a directory you preferred, then create new virtual python environment and activate it.
python -m venv chatbot-env
source chatbot-env/bin/activate
STEP 3: Install required packages
create a file called requirements.txt and add the openAI and dotenv packages.
openai==0.28.1
python-dotenv=1.0.0
Then install these packages using pip
.
pip install -r requirements.txt
STEP 4: Scripting
import os
import openai
from dotenv import load_dotenv
load_dotenv()
openai.api_key = os.getenv('OPENAI_API_KEY')
response = openai.ChatCompletion.create(
model="gpt-3.5-turbo",
messages=[
{"role": "system", "content": ""},
{"role": "user", "content": "Say 'Hello World'"}
],
temperature=0.7,
max_tokens=100,
)
response_message = response["choices"][0]["message"]
print(response_message)
Breakdown
- Import Required Packages
- Provide OpenAI API key to the script.
- use ChatCompletion.create method to send request to OpenAI.
- use gpt-3.5-turbo as the model
- message has to sections. first one is system definition and second one is user input.
- temperature controls the randomness of the response.
- max_token limit the response to 100 maximum tokens.
- print the response from OpenAI chatGPT.
Once you Execute the script you will get following output.
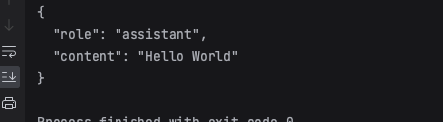
Alter the system definitions
Change the message block as follow to alter the behavior of the model.
messages=[
{"role": "system", "content": "Respond in french"},
{"role": "user", "content": "Say 'Hello World'"}
]
When this altered script run it will provide respons in French as follow.
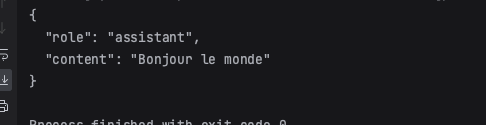
Change the message to give sentiment analysis behavior to the system. and observe the response.
messages=[
{"role": "system", "content": "Act as a sentiment analyzer"},
{"role": "user", "content": "I felt good today. I learn new thing about chatGPT"}
]
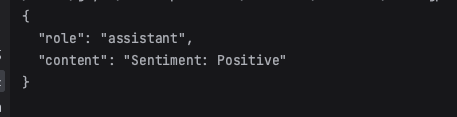
git repository: https://github.com/gayanzampaths/chat-gpt-hello-world
This is just the beginning of what you can do with ChatGPT. You can build more complex chatbots, automate customer support, or create virtual assistants to streamline various tasks.
To learn more about ChatGPT and its capabilities, check out the OpenAI documentation. Explore the possibilities and start building your own AI-powered conversational applications!